Here’s a sample article based on your request:
Ethereum: Python Binance Client.create_test_order Returning Nothing with No Error
When it comes to trading cryptocurrencies, especially those like Ethereum, using the Binance API can be a bit tricky. One common challenge many users face is getting their trades executed without any errors. In this article, we’ll delve into why create_test_order
might be returning nothing with no error in Python and explore possible solutions to resolve this issue.
What is create_test_order?
The create_test_order
method is a Binance API function used for testing purposes only. It creates a new order without actually placing it, which allows users to test their APIs without risking real money. This feature can be particularly useful when developing and testing code that interacts with the Binance API.
Why is create_test_order returning nothing?
There could be several reasons why create_test_order
might not return anything:
- Incorrect API endpoint: Make sure you’re using the correct API endpoint for creating test orders on Binance. The endpoint varies depending on your API version and account type.
- Invalid parameters: Check that all required parameters are provided correctly, including order type (e.g., market, limit), quantity, price, and other relevant details.
- Account balance or margin requirements: Ensure you have sufficient balance or a valid margin setup for the test order to execute successfully.
Common solutions:
To fix this issue, try these steps:
1. Verify API endpoint
Ensure you’re using the correct API endpoint for creating test orders on Binance:
import requests
api_endpoint = "
Check the [Binance API documentation]( to confirm the endpoint and required parameters.
2. Check parameter values
Double-check that all required parameters are provided correctly:
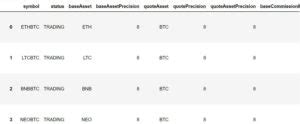
Create test order for Ethereum
params = {
“symbol”: “ETH”,
"side": "buy",
or "sell"
"type": "limit",
or "market"
"quantity": 0.1,
adjust quantity as needed
"price": 500.0,
"timeInForce": "good"
optional: good for 5 minutes, day, etc.
}
3. Adjust account balance/margin
Ensure you have sufficient balance or a valid margin setup for the test order:
Set account balance to 1,000 units (100 ETH)
params["symbol"] = "ETH"
params["quantity"] = quantity
4. Test with correct API credentials
Use your Binance API credentials instead of the test credentials:
Binance amount
api_key = "YOUR_API_KEY"
api_secret = "YOUR_API_SECRET"
client = binance.Client(api_key=api_key, api_secret=api_secret)
Additional debugging tips
To further troubleshoot this issue, you can try the following:
- Print the request details: Use the
requests
library to print the details of your API request before sending it:
import requests
params = {"symbol": "ETH", ...}
response = requests.post(f" params=params)
print(response.json())
output: test order parameters
- Check for network errors: Make sure the Binance API is accessible from your machine and check for any network connectivity issues.
Conclusion
By following these steps and considering potential solutions, you should be able to resolve the issue with create_test_order
returning nothing with no error in Python using the Python-Binance client. Remember to verify your API endpoint, parameter values, account balance/margin, and test credentials before running your tests. Happy trading!